Communication
- diagram
small talk
- How are you? Im doing great!
- Thank you for the interview!
- Have a good night! / Have a nice day!
interviewer
- can you hear me?
- can i assume that … ?
- walk through a small example / some test cases
- It looks good for me / Sounds good!
- Im not sure why you … ?
- in the line …
- can you analyze the time and space complexity?
- the question said that …
- there are some typo in your code
- clarify your idea
- I will be the interviewer fist
- make sense / make no sense
- on the right track
- you get the point!
- you can use either BFS or DFS
- Go ahead!
interviewee
- Let me give you a small example
- double check my code
- write some test cases to test my funciton
- as we can see
- analyze different cases first
- Lets say … variable …
- Can you share a small example for me?
- make sure that i understand the question!
Tree
- can we assume that class node has parent pointer?
Sales Path
Find the minimal sum cost from root to leaf in a Tree (Not necessarily binary)
1
2
3
4
5
6
7
8
9# Time : O(n), Space : O(n)
def get_cheapest_cost(rootNode):
if not rootNode.children:
return rootNode.cost
minCost = float('inf')
for child in rootNode.children:
minCost = min(minCost, get_cheapest_cost(child))
return minCost + rootNode.costfollow up:
TODO
BST Successor Search
In a Binary Search Tree (BST), an Inorder Successor of a node is defined as the node with the smallest key greater than the key of the input node (see examples below).
Given a node inputNode in a BST, you’re asked to write a function findInOrderSuccessor that returns the Inorder Successor of inputNode. If inputNode has no Inorder Successor, return null.
1 | def find_in_order_successor(self, inputNode): |
Case1 : inputNode has a right child. In this case successorNode would be the node with the minimum key in inputNode’s right subtree.
Case2: inputNode doesn’t have a right child. In this case successorNode would be one of inputNode’s ancestors. More specifically, within inputNode’s ancestor chain (starting from inputNode all the way up to the root), successorNode is the first parent that has a left child in that chain.
Other
H-Tree Construction
Write a function drawHTree that constructs an H-tree, given its center (x and y coordinates), a starting length, and depth. Assume that the starting line is parallel to the X-axis.
Use the function drawLine provided to implement your algorithm. In a production code, a drawLine function would render a real line between two points. However, this is not a real production environment, so to make things easier, implement drawLine such that it simply prints its arguments (the print format is left to your discretion).
Analyze the time and space complexity of your algorithm. In your analysis, assume that drawLine’s time and space complexities are constant, i.e. O(1).
1 | 1 |
Getting a Different Number
Given an array arr of unique nonnegative integers, implement a function getDifferentNumber that finds the smallest nonnegative integer that is NOT in the array.1
2
3
4
5
6
7
8# Time : O(n)
# Space : O(n)
def get_different_number(arr):
n = len(arr)
arr_set = set(arr)
for i in xrange(n+1):
if i not in arr_set:
return i
Array Index & Element Equality
Given a sorted array arr of distinct integers, write a function indexEqualsValueSearch that returns the lowest index i for which arr[i] == i. Return -1 if there is no such index.
- Binary Search
- Edge case
1 | # Time : O(logn) |
Number of Paths
Given n, the size of the grid’s axes, write a function numOfPathsToDest that returns the number of the possible paths the driverless car can take.
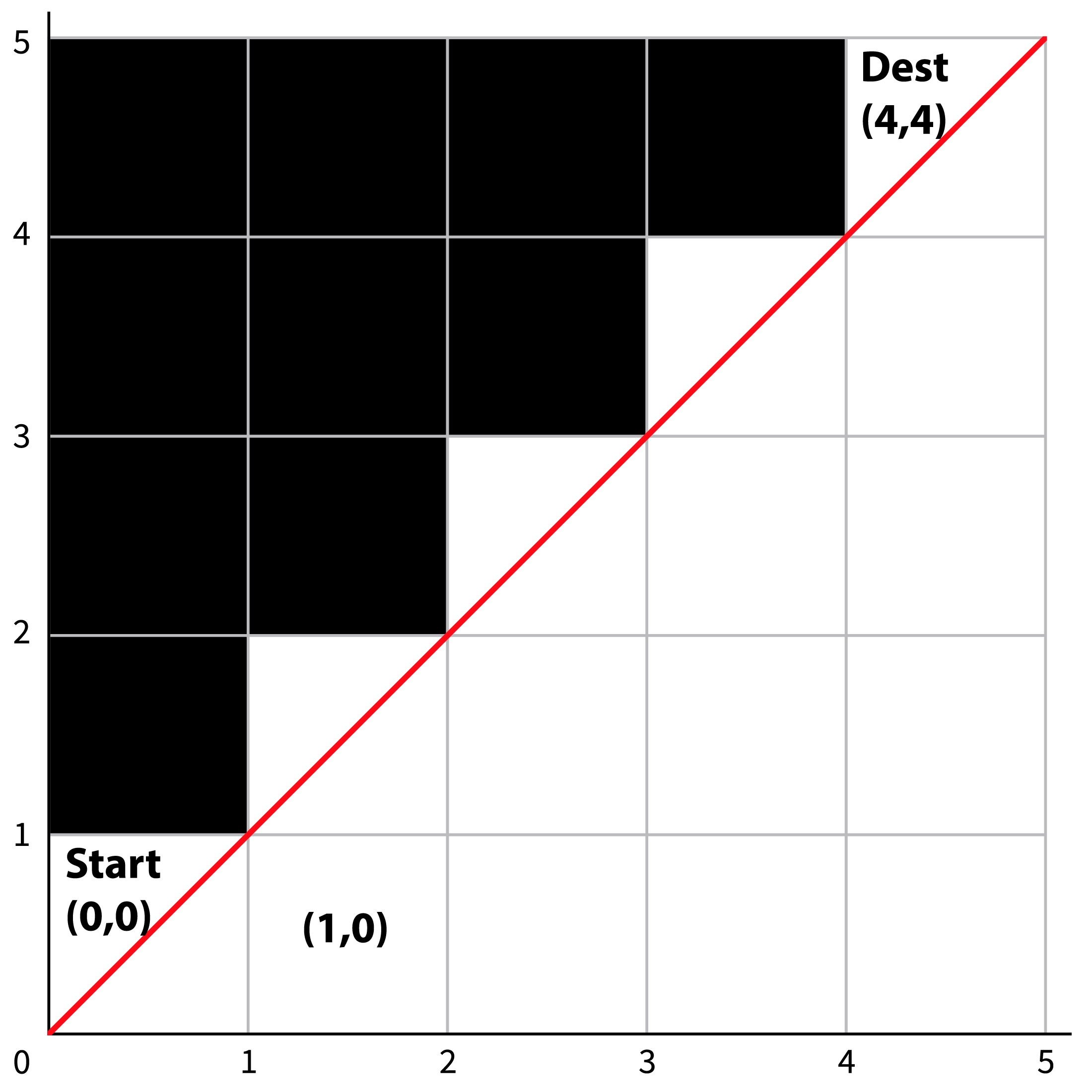
1 | def num_of_paths_to_dest(n): |
Explanation
Since the car can only move up and move down, when it arrives at a point, there are two possibilities:
- It arrives at the point from below cell (move up to the point)
- It arrives at the point from left cell (move right to the point)
Sentence Reverse
Solution1. python built-in function
1
2
3
4
5
6# Time : O(n), Spacce : O(n)
def reverse_words1(arr):
string = ''.join(arr)
chs = string.split()
res = ' '.join(chs[::-1])
return list(res)Solution2. Reverse Twice
- First: reverse all the word!
I love google -> I evol elgoog - Second: reverse the string character by charater
I evol elgoog -> google love I
1 | # Space O(n) -> O(1) |
Matrix Spiral Copy
Given a 2D array (matrix) inputMatrix of integers, create a function spiralCopy that copies inputMatrix’s values into a 1D array in a spiral order, clockwise. Your function then should return that array. Analyze the time and space complexities of your solution.
1 | class Solution: |